# Functions
This page is dedicated to the automation of the system: provide a mechanism of writing and creating one or more functions (PHP scripts), each one contaning user defined code.
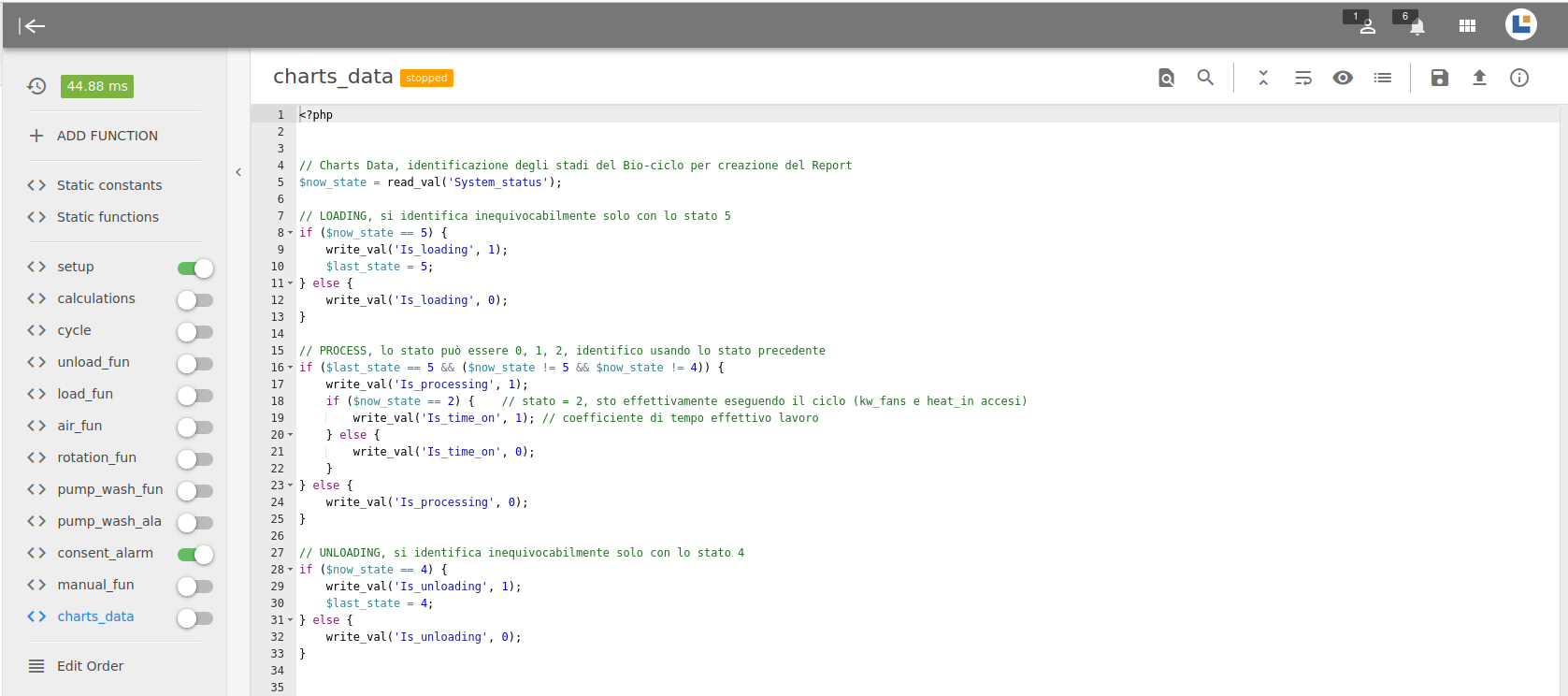
# Side panel
Functions classify into two categories, depending on how they are executed by PlexusLAB:
- Static constants and Static functions (first two on the left), they are executed only once at system reboot, in this sequence;
- All other functions are executed once every program cycle instead. Users decide how many of them are created, how they are defined and in what order they are executed (from top to bottom)
The configured function are listed on the left, with their name. The icon and the label take on color
- blue, to identify the functions currently selected and displayed in the view;
- gray, when function is turned on
The icon can be of two types and defines wheather the function is just saved or laso uploaded (more details below):
- code the function is the one actually executed by the system, not a draft version;
- file_upload the function displayed is a draft, its code has been modified but this differences are still not executed by the system. This occur when a modified functions is still not uploaded onto the executing system.
# Activating/Deactivating a function
A switch button appearing next to the function name define wheather related function is actually executed into program cycle or not.
- When a function is active, its status can be Running, when function is executed as expected, or Error an error occurred during execution;
- When a function is not active its code will be ignored. Its status is Not active
# Add a function
Adding a new function is done by clicking on the button labeled "ADD FUNCTION"; it opens a modal that allows to insert function name which must comply with the Plexus nomenclature criteria
# Re-ordering the functions
It is done by clicking on the "Edit Order" button at the bottom of the function list;
When clicked, a modal window is displayed that allows you to drag the rows and, if necessary, save the new order in order to see the list with the desired structure.
TIP
Note that the button is shown only when there are at least 2 functions configured.
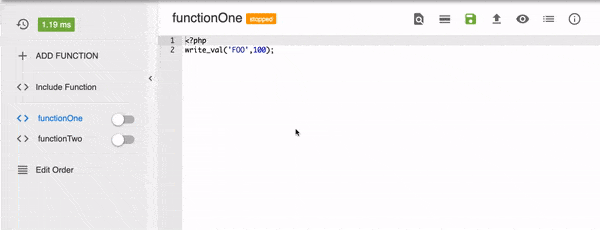
# Static constants
Using Static constants is suggested in order to define constants and global enumerative variables, so that they can be used inside othr functions.

# Static functions
The Static functions allows to declare custom API defined at global level that can be used inside other functions. Some examples are shown into Appendices page. Global functions are created by code developers in order to complete specific tasks whenever they need to be executed frequently and require several lines of code. Global functions helps to keep a clean and more understandable code structure.

# View
# Function Top Bar
Every function have a function top bar that allows user to perform various actions on the functions.
Icon | Name | Information |
---|---|---|
find_in_page | Search in Functions | An action that allows user to search specific variables in all of your functions; non-case-sensitive |
search | Search in Devices/Sections | Allows user to search for a word into Sections/Devices; non-case-sensitive |
calendar_view_day | Fold / Unfold Code | An action that allows you to minimized the code |
wrap_text | Align | Align code |
remove_red_eye | Debug Messages | An action that allows user to read error message. Similiar like console log. |
list | API List | A library of API List function to use. |
save | Save | An action that save the code after you edit. Function won't run in the newest code until you uploaded it even though you saved. |
upload | Upload | An action that upload the code after you saved. Function won't run in the newest code until you uploaded it even though you saved. |
info_outline | Info | Allow user to edit the function name and delete it. |
# Writing and Executing Functions
There are Four steps to write and execute your functions
- Create a function;
- Write the code (refer to our API List in order to know PlexusLAB default functions, others are PHP default functions, version 7 or 8 according to installed version);
- Save the function;
- Upload the function;
- Activate the function
# Autocomplete
The code editor comes with an autocomplete feature that helps to find names of variables, channels, functions and constants.
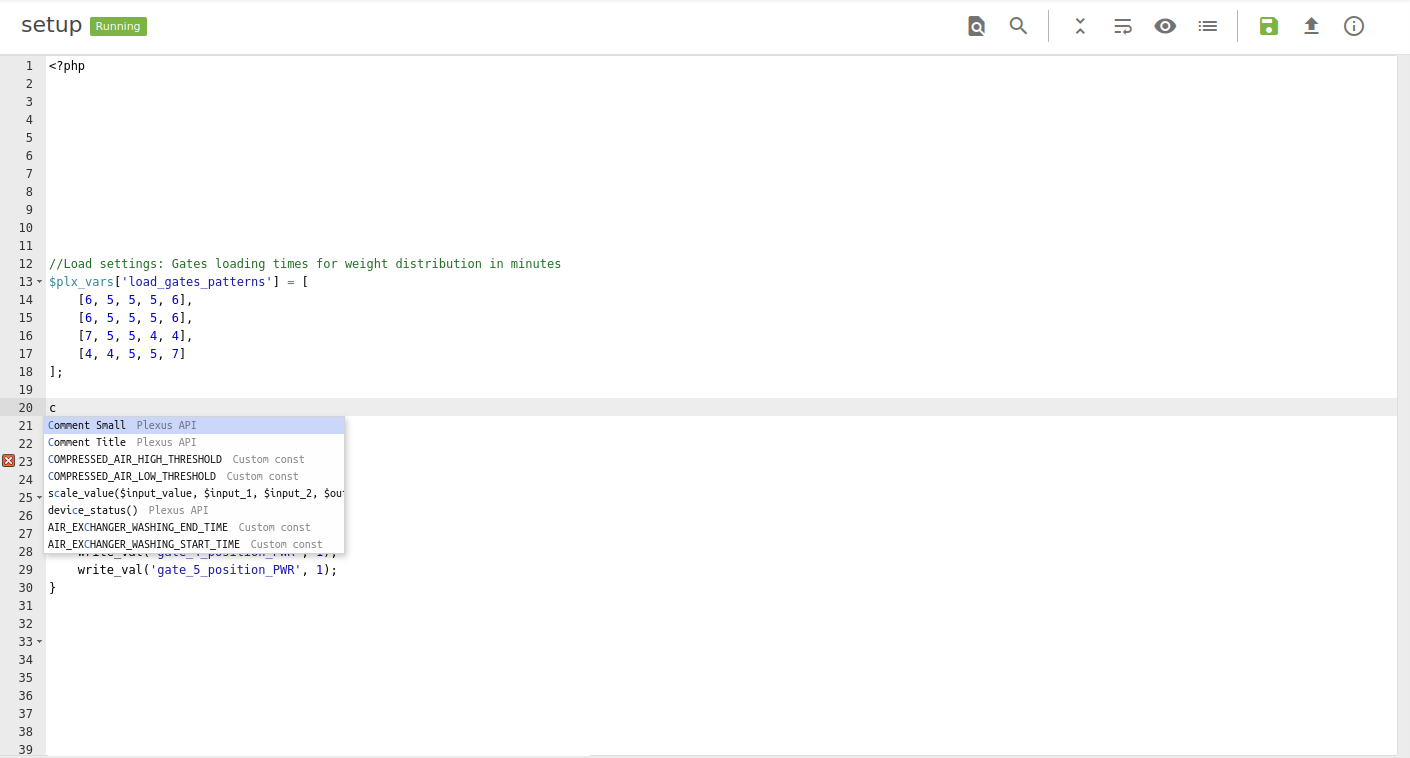
# Rules for Functions
Important Rules
- Code language is PHP;
- Writing functions script must be comply with the Plexus nomenclature criteria;
- While loop will cause the code to crash due to Ladder Logic;
- Using variable that you created from Section page does not require '$';
- Functions are execute from top to bottom order, functions that are below will overwrite any functions that are on top if they write towards the same variable for example;
- Turning off functions does not revert back value that have being set!;
- Do note, in order to read and write specific device channels, devices have to be turned ON
# Functions Library API
General APIs
if / Read
if / Read / Write
Comment Title
Comment Small
debug_message('message')
info_message('message', 'INFO')
function_active('function_id')
is_sensor_faulty('channel_name')
all_faulty_sensors()
Variable APIs
read_val('var_name')
read_future_val('var_name', $seconds_in_the_future, $seconds_in_the_past)
write_val('var_name', $value)
write_val_delay('var_name', $value, $msDelay)
reset_val_delay('var_name')
write_val_period('var_name', $value1, $value2, $ms1, $ms2)
reset_val_period('var_name')
write_val_ramp('var_name', $rampValueStart, $rampValueEnd, $msRampDuration)
reset_val_ramp('var_name')
write_val_at_time('var_name', $value, '16:20')
write_val_at_time_range('var_name', $value, '16:20', '16:30')
disable('var_name')
enable('var_name')
get_bounded_value($input_value, $min_value, $max_value)
is_value_inside_target_range($input_value, $target_value, $offset = null)
is_value_inside_target_range_mod($input_value, $input_min, $input_max, $target_value, $offset = null)
scale_value($input_value, $input_1, $input_2, $output_1, $output_2, $round = true)
to_bitmap($values, $lsb_first = true, $signed = false)
from_bitmap($bitmap, $length = 16)
increment_cycling_variable($var_name, $max_value_before_reset, $var_reset_value = 0)
watchdog_on_bit_inversion($watchdog_timer_name, $watchdog_timeout_var, $watchdog_actual_value)
moving_average('var_name', $window_in_samples)
reset_moving_average('var_name', $window_in_samples)
fixed_average('var_name', $window_in_seconds)
reset_fixed_average('var_name', $window_in_seconds)
moving_max('var_name', $window_in_seconds)
reset_moving_max('var_name', $window_in_seconds)
moving_min('var_name', $window_in_seconds)
reset_moving_min('var_name', $window_in_seconds)
Timers APIs
start_timer('timer_name')
reset_timer('timer_name')
pause_timer('timer_name',1)
timer_running('timer_name')
read_timer('timer_name')
set_timer_duration('timer_name', $duration)
Alert APIs
set_alert('alert_name', 'message', ['subordinated_alert_name_1', ...])
set_alert_delay('alert_name', 'message', $msDelay, ['subordinated_alert_name_1', ...])
reset_alert('alert_name')
alert_active('alert_name')
any_alert_active()
Stepper APIs
start_step('step_name')
read_step('step_name')
set_step('step_name', $value)
PID control APIs
PID_controller('PID_name', $PID_params)
reset_PID_controller('PID_name')
PWM_controller('var_name', $duty, $msPeriod)
reset_PWM_controller('var_name')
Flow APIs
is_interval_of($name, $interval_in_seconds)
since_startup()
delay_action()
reset_delay_action()
Filtering APIs
IIR_2o_LP('var_name', pole)
reset_IIR_2o_LP
List of each Functions API in detail are available here.